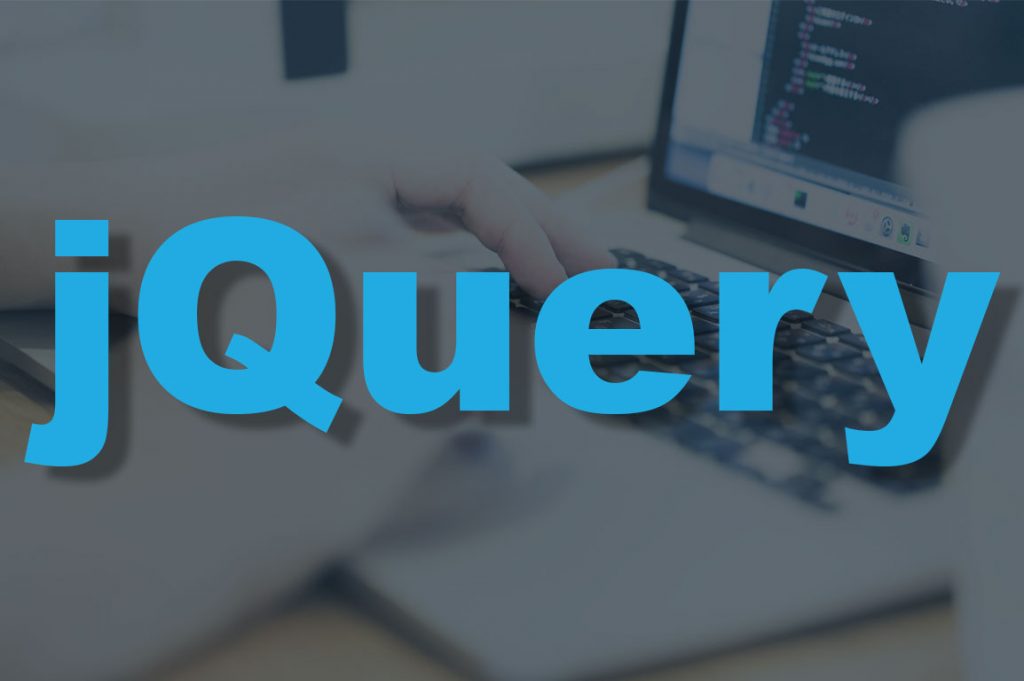
どうも、べ〜やんです。
今回は、jQueryを使ってハンバーガーメニューを作る方法を紹介します。

目次
ハンバーガーメニューとは
ハンバーガーメニューとは、例えばヘッダーのメニューを隠しておいてアイコンをクリックするとメニューが表示させたりするもので、Webページをスマートフォンなどで見たときによく使われます。
See the Pen XWWqpro by beeyan (@orientado) on CodePen.
作り方
簡単な作り方をざっくり紹介します。
HTMLでメニューとアイコンを用意。
↓
CSSで見た目を整えてメニューを見えなくしておく。
↓
jQueryでアイコンをクリックすると動き出すように設定。
HTML
使用するHTMLは以下の通り
アイコンはborder
でデザインするので中身は空です。
<!-- ナビメニュー -->
<nav class="menu">
<ul class="menu__ul">
<li class="menu__ul--item"><a href="#">メニュー1</a></li>
<li class="menu__ul--item"><a href="#">メニュー2</a></li>
<li class="menu__ul--item"><a href="#">メニュー3</a></li>
<li class="menu__ul--item"><a href="#">メニュー4</a></li>
<li class="menu__ul--item"><a href="#">メニュー5</a></li>
</ul>
</nav>
<!-- アイコン -->
<div class="icon">
<span></span>
<span></span>
<span></span>
</div>
CSS
.menu {
position: fixed;
top: 0;
right: 0;
text-align: center;
width: 100%;
transform: translateY(-100%);
transition: all 0.8s;
z-index: 2;
}
position: fixed;
で位置を固定。
位置はtop: 0; right: 0;
で右上を起点にしています。
transform: translateY(-100%);
で上に100%移動させて隠しています。
z-index: 2;
で他の要素より上に来るように重なり順を設定。
transition: all 0.8s;
ナビが動くスピードを設定。
.menu__ul {
width: 100%;
margin: 0 auto;
padding: 0;
background: skyblue;
}
メニューリストの色や大きさを指定。
.menu__ul--item {
list-style-type: none;
padding: 0;
width: 100%;
border-bottom: 1px solid #333;
}
list-style-type: none;
でリストの点を消す。
border-bottom: 1px solid #333;
下線を指定。
.menu__ul--item a {
display: block;
padding: 0.5em 0;
color: #fff;
text-decoration: none;
}
display: block;
クリック出来る範囲を要素全体に広げる。
text-decoration: none;
でリンクの下線を非表示。
.active {
transform: translateY(0%);
}
メニューにactiveクラスを付け加えた時の設定。
transform: translateY(0%);
でクリック後のメニューの位置を指定。
activeクラス
はHTMLには記述しません。jQueryで後から追加します。
.icon {
display: block;
position: fixed;
top: 10px;
right: 10px;
width: 40px;
height: 40px;
cursor: pointer;
z-index: 3;
}
アイコンの位置を指定。
cursor: pointer;
でカーソルが変化してクリック出来るとわかるようにする。
z-index: 3;
で重なり順をメニューより上に。
.icon span {
display: block;
position: absolute;
width: 30px;
border-bottom: solid 2px;
-webkit-transition: .30s;
-moz-transition: .30s;
transition: .30s;
}
iconのspanにborder-bottom: solid 2px;
を指定します。
これがハンバーガーメニューのアイコンの3本線になります。
transition: .30s;
で3本線が×になるまでのスピードを指定。
.icon span:nth-child(1) {
top: 10px;
}
.icon span:nth-child(2) {
top: 20px;
}
.icon span:nth-child(3) {
top: 30px;
}
3本線のそれぞれの位置を指定。
.active span:nth-child(1) {
top: 20px;
-webkit-transform: rotate(-45deg);
-moz-transform: rotate(-45deg);
transform: rotate(-45deg);
}
.active span:nth-child(2) {
top: 20px;
-webkit-transform: rotate(45deg);
-moz-transform: rotate(45deg);
transform: rotate(45deg);
}
.active span:nth-child(3) {
top: 20px;
-webkit-transform: rotate(45deg);
-moz-transform: rotate(45deg);
transform: rotate(45deg);
}
iconの要素にactiveクラスを付け加えた時の設定。
1本目の線をtransform: rotate(-45deg);
で傾ける。
2本目3本目はtransform: rotate(45deg);
で一本目と反対方向に傾ける。
2本目と3本目の設定が全く同じなので2本の線が重なって一本に見えるようになる。
CSS全体↓
.menu {
position: fixed;
top: 0;
right: 0;
text-align: center;
width: 100%;
transform: translateY(-100%);
transition: all 0.8s;
z-index: 2;
}
.menu__ul {
width: 100%;
margin: 0 auto;
padding: 0;
background: skyblue;
}
.menu__ul--item {
list-style-type: none;
padding: 0;
width: 100%;
border-bottom: 1px solid #333;
}
.menu__ul--item a {
display: block;
padding: 0.5em 0;
color: #fff;
text-decoration: none;
}
.active {
transform: translateY(-7%);
}
.icon {
display: block;
position: fixed;
top: 10px;
right: 10px;
width: 40px;
height: 40px;
cursor: pointer;
z-index: 3;
}
.icon span {
display: block;
position: absolute;
width: 30px;
border-bottom: solid 2px;
-webkit-transition: .30s;
-moz-transition: .30s;
transition: .30s;
}
.icon span:nth-child(1) {
top: 10px;
}
.icon span:nth-child(2) {
top: 20px;
}
.icon span:nth-child(3) {
top: 30px;
}
.active span:nth-child(1) {
top: 20px;
-webkit-transform: rotate(-45deg);
-moz-transform: rotate(-45deg);
transform: rotate(-45deg);
}
.active span:nth-child(2) {
top: 20px;
-webkit-transform: rotate(45deg);
-moz-transform: rotate(45deg);
transform: rotate(45deg);
}
.active span:nth-child(3) {
top: 20px;
-webkit-transform: rotate(45deg);
-moz-transform: rotate(45deg);
transform: rotate(45deg);
}
jQuery
$(function() {
$('.icon').click(function() {
$(this).toggleClass('active');
if ($(this).hasClass('active')) {
$('.menu').addClass('active');
} else {
$('.menu').removeClass('active');
}
});
});
$('.icon').click(function()
アイコンをクリックした時動き出すように指定。
$(this).toggleClass('active');
でiconの要素にactiveクラスをつけたり外したりする。
if ($(this).hasClass('active'))
はiconの要素がactiveクラスを持っているかどうかを判定。
$('.menu').addClass('active');
でmenuクラスにもactiveクラスをつける。
else
はif
と反対だった時の指定。
$('.menu').removeClass('active');
でactiveクラスを外す。
つまりiconの要素がactiveクラスを持つかどうかをクリックで切り替える、iconの要素がactiveクラスを持っていればmenuクラスにもactiveクラスを与え、iconの要素がactiveクラスを持っていなければmenuクラスもactiveクラスを外す指定。
おわりに
今回は、jQueryを使ってハンバーガーメニューを作る方法を紹介しました。
レスポンスデザインの時などに便利ですね。
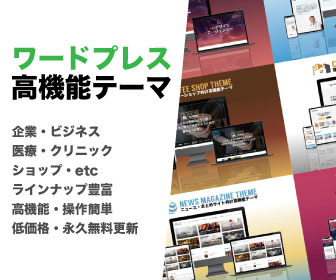